Creating predictive maps using Machine Learning (ML) in Python and visualizing them with Leaflet is a powerful way to leverage spatial data for predictive analysis. This process involves using spatial data in a machine learning model to predict a geographic outcome, followed by visualizing the prediction results on an interactive web map using Leaflet.
The ML for Predictive Maps in Python and Leaflet course includes all of the most recent information to keep you abreast of the employment market and prepare you for your future. The curriculum for this excellent ML for Predictive Maps in Python and Leaflet course includes modules at all skill levels, from beginner to expert. You will have the productivity necessary to succeed in your organisation once you have completed our Machine Learning for Predictive Maps in Python and Leaflet Program.
Machine Learning
Machine learning (ML) is a branch of artificial intelligence (AI) and computer science that focuses on the using data and algorithms to enable AI to imitate the way that humans learn, gradually improving its accuracy. The three (3) main parts of machine learning include:
- Decision Process: In general, ML algorithms are used to make a prediction or classification. Based on some input data, which can be labeled or unlabeled, your algorithm will produce an estimate about a pattern in the data.
- Error Function: An error function evaluates the prediction of the model. If there are known examples, an error function can make a comparison to assess the accuracy of the model.
- Model Optimization Process: If the model can fit better to the data points in the training set, then weights are adjusted to reduce the discrepancy between the known example and the model estimate. The algorithm will repeat this iterative “evaluate and optimize” process, updating weights autonomously until a threshold of accuracy has been met.
By enroling in ML for Predictive Maps in Python and Leaflet, you can kickstart your vibrant career and strengthen your profound knowledge. You can learn everything you need to know about the topic. So enrol in our ML for Predictive Maps in Python and Leaflet course right away if you’re keen to envision yourself in a rewarding career.
Description of Machine Learning
Enroling in this ML for Predictive Maps in Python and Leaflet course can improve your ML for Predictive Maps in Python and Leaflet perspective, regardless of your skill levels in the ML for Predictive Maps in Python and Leaflet topics you want to master. If you’re already a Machine Learning for Predictive Maps in Python and Leaflet expert, this peek under the hood will provide you with suggestions for accelerating your learning, including advanced Machine Learning for Predictive Maps in Python and Leaflet insights that will help you make the most of your time. This Machine Learning for Predictive Maps in Python and Leaflet course will act as a guide for you if you’ve ever wished to excel at Machine Learning for Predictive Maps in Python and Leaflet.
Why Machine Learning?
-
- This course is accredited by the CPD Quality Standards.
-
- Lifetime access to the whole collection of the learning materials.
-
- Online test with immediate results.
-
- Enroling in the course has no additional cost.
-
- You can study and complete the course at your own pace.
-
- Study for the course using any internet-connected device, such as a computer, tablet, or mobile device.
Who is this course for?
This ML for Predictive Maps in Python and Leaflet course is a great place to start if you’re looking to start a new career in Machine Learning for Predictive Maps in Python and Leaflet field. This training is for anyone interested in gaining in-demand ML for Predictive Maps in Python and Leaflet proficiency to help launch a career or their business aptitude.
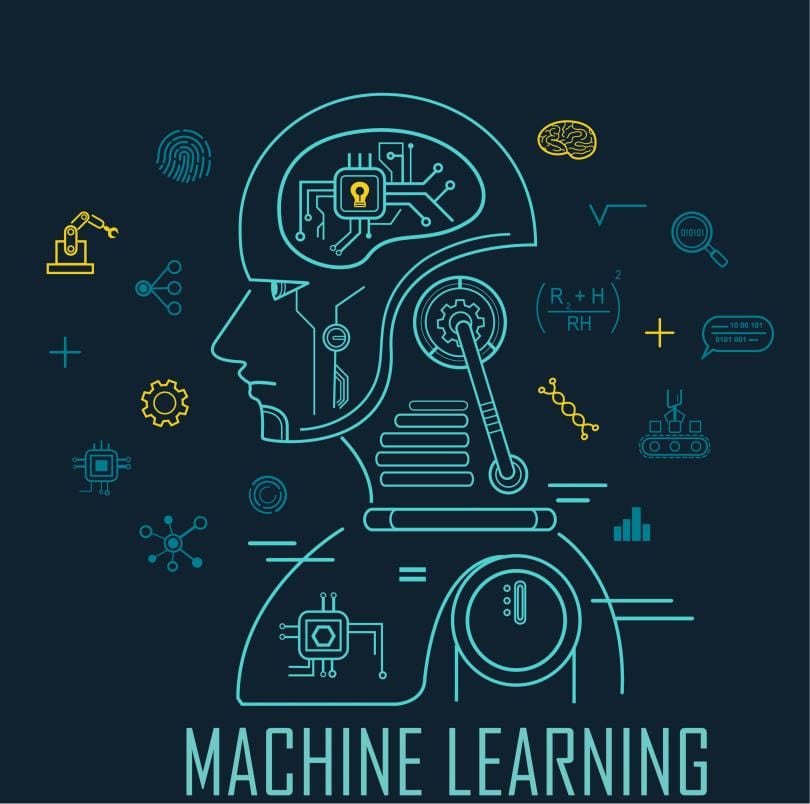
Requirements
The ML for Predictive Maps in Python and Leaflet course requires no prior degree or experience. All you require is English proficiency, numeracy literacy and a gadget with stable internet connection. Learn and train for a prosperous career in the thriving and fast-growing industry of Machine Learning for Predictive Maps in Python and Leaflet, without any fuss.
Career Path
This ML for Predictive Maps in Python and Leaflet training will assist you develop your Machine Learning for Predictive Maps in Python and Leaflet ability, establish a personal brand, and present a portfolio of relevant talents. It will help you articulate a Machine Learning for Predictive Maps in Python and Leaflet professional story and personalise your path to a new career. Furthermore, developing this Machine Learning for Predictive Maps in Python and Leaflet skillset can lead to numerous opportunities for high-paying jobs in a variety of fields.
Course Curriculum
Section 01: Introduction
Section 02: Setup and Installations
Section 03: Writing the Django Server-Side Code
Section 04: Writing the Application Front-end Code
Section 05: Machine Learning
Section 06: Automating the Machine Learning Pipeline
Section 07: Leaflet Programming
Section 08: Project Source Code
Steps of creating predictive maps
Step 1. Prepare Spatial Data for Machine Learning
- Data Collection: Gather spatial data relevant to the prediction task, such as environmental data, demographic data, or historical data (e.g., land use, terrain elevation, or rainfall patterns).
- Spatial Data Processing: Use geospatial libraries in Python like GeoPandas and Shapely to handle spatial data and extract useful features for machine learning.
- GeoPandas is useful for working with vector data, loading shapefiles, and converting them into tabular data with spatial attributes.
- Ensure data is in a suitable projection (e.g., UTM) to allow accurate analysis.
import geopandas as gpd
# Load spatial data (e.g., shapefile or GeoJSON)
gdf = gpd.read_file(‘path_to_your_data.shp’)
# Explore the data and extract features (e.g., elevation, land cover, etc.)
print(gdf.head())
- Extract Features: Create a feature set that can be fed into a machine learning model. This may include spatial variables such as proximity to roads, elevation, land use, etc.
- Target Variable: Identify the outcome you want to predict (e.g., flood risk, land suitability, etc.) and add it to the dataset.
Step 2: Build a Machine Learning Model in Python
Use libraries such as scikit-learn or TensorFlow to build a machine learning model.
- Preprocess Data: Split your data into training and testing sets and preprocess it for use in machine learning (e.g., normalize the data, handle missing values).
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
# Features and target
X = gdf.drop(columns=[‘target’, ‘geometry’]) # Features
y = gdf[‘target’] # Target (prediction variable)
# Train-test split
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# Scale the data
scaler = StandardScaler()
X_train_scaled = scaler.fit_transform(X_train)
X_test_scaled = scaler.transform(X_test)
- Train Model: Fit an appropriate machine learning model (e.g., Random Forest, Decision Tree, or Linear Regression).
from sklearn.ensemble import RandomForestRegressor
# Instantiate and train the model
model = RandomForestRegressor(n_estimators=100, random_state=42)
model.fit(X_train_scaled, y_train)
# Make predictions
predictions = model.predict(X_test_scaled)
- Evaluate the Model: Use performance metrics to evaluate your model, such as mean squared error for regression tasks or accuracy for classification tasks.
from sklearn.metrics import mean_squared_error
# Evaluate model performance
mse = mean_squared_error(y_test, predictions)
print(f”Mean Squared Error: {mse}”)
3. Make Predictions for Spatial Data
After training your model, make predictions for the entire spatial dataset or for new data points across the region of interest.
- Apply the trained model to the entire dataset or a grid of spatial points, generating predictions that can be visualized.
# Apply model to full dataset or new points
gdf[‘predictions’] = model.predict(scaler.transform(X))
4. Convert Predictions into GeoJSON for Leaflet
To visualize the predictions on a Leaflet map, you need to convert your results into GeoJSON format. GeoJSON is a common format for representing geographic data that Leaflet can easily consume.
# Save predicted values into GeoJSON format
gdf.to_file(“predictions.geojson”, driver=”GeoJSON”)
- Each feature in the GeoJSON should include the geometry and the prediction value as part of the properties. This allows you to style the map based on the predictions.
5. Visualize Predictive Maps in Leaflet
Leaflet is a lightweight JavaScript library for interactive maps. To visualize the predictive maps, you can create a Leaflet web map and load the GeoJSON predictions into it.
- Create a basic Leaflet map and load the GeoJSON data using the
L.geoJSON()
method. - Use color scales to represent the predicted values visually.
Here’s an example of how to set up the Leaflet map:
<!DOCTYPE html>
<html>
<head>
<title>Predictive Map</title>
<meta charset=”utf-8″ />
<meta name=”viewport” content=”width=device-width, initial-scale=1.0″>
<link rel=”stylesheet” href=”https://unpkg.com/leaflet/dist/leaflet.css” />
<style>
#map { height: 600px; }
</style>
</head>
<body>
<div id=”map”></div>
<script src=”https://unpkg.com/leaflet/dist/leaflet.js”></script>
<script>
// Initialize the map
var map = L.map(‘map’).setView([latitude, longitude], zoom_level);
// Add a base map layer (e.g., OpenStreetMap)
L.tileLayer(‘https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png’, {
attribution: ‘© OpenStreetMap contributors’
}).addTo(map);
// Load the GeoJSON prediction data
fetch(‘predictions.geojson’)
.then(response => response.json())
.then(data => {
// Define a color scale based on prediction values
function getColor(prediction) {
return prediction > threshold1 ? ‘#800026’ :
prediction > threshold2 ? ‘#BD0026’ :
prediction > threshold3 ? ‘#E31A1C’ :
prediction > threshold4 ? ‘#FC4E2A’ :
‘#FFEDA0’;
}
// Style function for GeoJSON
function style(feature) {
return {
fillColor: getColor(feature.properties.predictions),
weight: 2,
opacity: 1,
color: ‘white’,
dashArray: ‘3’,
fillOpacity: 0.7
};
}
// Add GeoJSON layer with the predictions
L.geoJSON(data, {style: style}).addTo(map);
});
</script>
</body>
</html>
Technologies used
- Python Libraries:
- GeoPandas: For spatial data manipulation and feature extraction.
- scikit-learn or TensorFlow: For building machine learning models.
- Pandas: For data manipulation and preprocessing.
- Matplotlib or Seaborn: For initial data visualization (optional).
- Leaflet.js:
- A lightweight JavaScript library to create interactive maps for the web.
- Works well with GeoJSON, allowing easy integration with predictive spatial data.